Intro to JavaScript
Designing with Web
Things are about to get even more fun!
JavaScript (JS for short) is the only programming language we'll see during this course. Created in 1995, JS rapidly imposed itself as the standard and is now the programming language of the Web.
Why is it interesting to learn JS?
Created to add dynamism and interactions by manipulating or creating HTML elements in a web page, JS can now be used to know what links your users click on or interactions they have with your pages. But we also can create games, data visualization, music, motion design, physics simulations, computer vision, 3D or even virtual reality, and much more…
Web pages are not the only place where JavaScript is used. You can automate your workflow on all Google Apps. Many desktop apps like Slack, Skype and more, are made with JS and web techs. JS engines grew up so much that you can now control robotics and IOT. Some databases, like MongoDB and CouchDB, also use JS as their programming language.
All in all, JS has exploded in popularity and is now a very useful skill to learn.
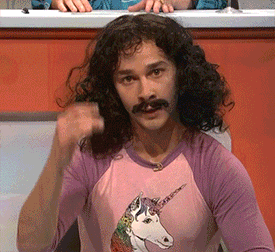
JavaScript and Java are completely different languages, both in concept and design, here we're only talking about JS.
How to use JS?
Like CSS, there are several options to use JS with our pages. The simplest one is to create a script
element that directly contains JS code, this is what we're going to start with:
<script>
alert( "Hello JS" ); // this instruction opens a popup
</script>
But like for CSS, it is better to create a script
elements with an src
attribute that points to a .js
file:
<script src="myScript.js"></script>
// where myScript.js is a file containing:
// alert( "Hello JS" );
As you may have understood, in JS everything after //
is a comment. We can also do multi-line comments starting by /*
and ending by */
, everything between these is comments, exactly the same way we write CSS comments.
// this is a single line comment
/*
this is a
multi-lines comment
*/
Here is a first example of JavaScript manipulating HTML elements, it replaces the content of a span
element with the date at the time the example is loaded:
Our script
element contains 2 instructions:
-
var dateHolder = document.querySelector( "#date" );
creates a variable (we'll see what that means later) that selects the element withid="date"
, just like CSS selectors. -
dateHolder.innerHTML = new Date();
sets the text of our element to the current date
The reason we've put the script
element at the end of the body
is that an HTML document is created by the browser in the order elements appear in the file. If the JavaScript is read before the HTML elements it is supposed to affect, it won't work since these elements are not yet created by the browser.
JS code is executed as soon as it is read by the browser.
Writing JS
Let's explain some of the core features of the JavaScript language, to get a better understanding of how it all works. It is worth noting that these features are common to all programming languages, so if you master these fundamentals, you're well on your way to being able to program just about anything!
For this we'll have a look at:
- Variables: containers to store values
- Conditions: take decisions with code
- Repetition loops: repeat an action multiple times
- Functions: modules to re-use code
- Arrays: store multiple values
- Objects: store complex data structures and more
Instead of using Glitch and manipulating HTML elements, we're going to use repl.it embeds, a simple and interactive way for us to experiment with these different programming concepts.
So, instead of having a web page for result, we're going to use a JavaScript console and the console.log();
instruction to print results in it.
Make sure to run and understand the content of these embeds by manipulating the code, i.e. writing code.
We need to click on the run button to execute the content of our script and see the result printed (do not pay attention to the => undefined
).
Try it:
A semicolon ;
at the end of a line indicates where an instruction ends, it is only absolutely required when you need to separate statements on a single line. However, some people believe that it is a good practice to put them in at the end of each statement. There are other rules for when you should and shouldn't use them — see Your Guide to Semicolons in JavaScript for more details.
#ProTip: You can also press Ctrl
+Enter
(Windows) / Cmd
+Enter
(Mac) to run the script.
All browsers have a JS console to test code / print informations and errors for developers:
- on Chrome, press
Ctrl
+Shift
+J
(Windows) /Cmd
+Opt
+J
(Mac) - on Firefox, press
Ctrl
+Shift
+K
(Windows) /Cmd
+Opt
+K
(Mac)
Let's start with variables.