JS - Libraries
Designing with Web
Libraries to the rescue!
What are libraries?
Remember CSS frameworks providing pre-written solutions for styling our pages? There also exist this kind of open-source tools for JavaScript: JS Libraries.
Libraries are collections of functions and variables and other JS bits we've seen previously, and each are specialized for one particular domain. There exist libraries for everything that can be done with JS, from manipulating images to controlling micro-controllers. JS libraries are compilation of JavaScript code that we can use without rewriting it.
We're going to have a look at the most widely used library on the web:

Hello jQuery
jQuery takes a lot of common tasks that require many lines of JavaScript code to accomplish, and wraps them into methods that you can call with a single line of code, like:
- HTML/DOM manipulation: create or remove elements, retrieve or modify their content
- CSS manipulation: modify the style of our elements
- HTML event methods: call functions when a user interacts with our pages
- Effects and animations: create transitions for our elements
- AJAX: for loading data
- And more…
Tip: In addition, jQuery has plugins for almost any task out there, from creating background with video to carousels or menus.
Just like CSS frameworks, to use a JS library like jQuery, we first need to include it in our document, with a few differences:
-
instead of a
link
, we are going to use ascript
element, since this is a JS file. -
instead of including it in the
head
of our document, we'll put it at the end of thebody
of our pages with our other scripts.
Stylesheets are usually put in the head
in order to be loaded before the browser renders our page and then renders elements as we want, while JS scripts are usually put at the end of the body
so that the browser renders our page first then loads our scripts.
We can load jQuery from CDNjs, a service that host thousands of libraries for us, as well as CSS frameworks (actually we already used it for loading normalize.css
and Skeleton
):

CDNs can offer a performance benefit by hosting jQuery on servers spread across the globe. This also offers an advantage that if the visitor to your webpage has already downloaded a copy of jQuery from the same CDN, it won't have to be re-downloaded.
So at the end of our document's body, we can include jQuery:
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
The jQuery library exposes a single object, with a lot of methods, that can be accessed using the variable name jQuery
or its shorter alias: $
As you may remember from variables naming rules, $
and _
(underscore) are the 2 only special characters that can be used in variable names.
jQuery works with selectors just like CSS to target specific elements, i.e. $( "#title" )
will select the element with the title
id, and wraps it in a JS object with jQuery methods like $( "#title" ).text()
to get its text, or $( "#title" ).text( "hello jQuery!" )
to set its text:
Uncomment line 23 (remove <!--
and -->
) to include main.js
to our page, then look at the code in main.js
and observe how it modifies the content of our page.
Reminder: You can see the results of console.log()
by opening your browser's Console:
- on Chrome, press Ctrl
+Shift
+J
(Windows) / Cmd
+Opt
+J
(Mac)
- on Firefox, press Ctrl
+Shift
+K
(Windows) / Cmd
+Opt
+K
(Mac)
If you open the Console here, you should see a lot of messages/errors since there are a lot of embeds and third parties scripts. In order to clearly read console messages for glitch embeds, right click on the Edit button → Open in a new tab to open the glitch editor (make modifications in the code there if needed), then click on Show live and finally open Console as mentioned above.
Note: If we need to use several methods on the same elements, instead of having jQuery search through our document each time, we should store the object created by jQuery in a variable to reuse it:
// we select all the elements with the "important" class and store that in a variable
var importants = $( ".important" );
// now we can use its methods
importants.text( "this is a paragraph" );
importants.css( "color", "red" );
// or we can chain methods:
// importants.text( "this is a paragraph" ).css( "color", "red" );
You might think "Ok, so we can create elements, change their text, apply styling, etc… but nothing that couldn't be done directly with HTML or CSS". Let's change that with more dynamic examples.
Passing functions
With jQuery's methods, we can also pass a function that returns a value, instead of a fixed value (cf. "this is a paragraph"
or "red"
in the previous note):
Note: for simplicity of the example I'm not creating a complete HTML document (doctype
/head
/body
) which is totally fine for demos and tests, but must be avoided otherwise.
Read more about the JavaScript Date and its methods to work with dates.
In this example we are creating a getCurrentDate
function; but if we don't need to reuse this function, we could also have written this:
$( "#date" ).text( function() {
var date = new Date();
return date.toLocaleDateString();
} );
When passing a function as argument we can also have access to the element on which the function is executed using this
(remember JS Objects?):
Listening for events
jQuery also provides an on
method to add interaction by listening to events on elements, for example changing the size of a paragraph when the value of a slider changes:
You can find the complete list of event types on MDN.
Or we could do a simple task manager:
Good job! This is our first app! Without the pre-added tasks and all the comments, we have a simple yet functionnal task manager with 11 lines of JS! 🔥
Before letting you explore jQuery on your own, there is one last functionality of jQuery that we need to study: loading data.
Loading data & dynamic content
We've talked about data formats like CSV, XML and JSON in previous parts. JSON is the most used nowadays because of its many advantages such as being lighter and a part of JavaScript.
In JS - Objects, we had this example:
We can store the value of authors
in a authors.json
file, we just need to put the name of the keys inside double quotes to make it valid JSON:
[
{
"name": "H. P. Lovecraft",
"birthDate": "20/08/1890",
"dateOfDeath": "15/03/1937",
"books": [
{
"title": "The Rats in the Walls",
"year": "1924"
},
{
"title": "The Call of Cthulhu",
"year": "1928"
}
]
},
{
"name": "J. R. R. Tolkien",
"birthDate": "01/03/1892",
"dateOfDeath": "09/02/1973",
"books": [
{
"title": "The Hobbit",
"year": "1937"
},
{
"title": "The Lord of the Rings",
"year": "1954"
}
]
},
{
"name": "Philip K. Dick",
"birthDate": "12/16/1928",
"dateOfDeath": "03,02,1982",
"books": [
{
"title": "Do Androids Dream of Electric Sheep?",
"year": "1968"
},
{
"title": "Ubik",
"year": "1969"
}
]
}
]
jQuery provides a .getJSON()
method to load a json file and execute a function once the data is loaded. This method takes 2 arguments:
- the path to the json file
-
a callback function to execute once the data is loaded. This function will gives us a
myDataset
argument containing the whole dataset.
Today thousands of services provide access to their data in JSON format, let's have a look at Random User Generator, a service that generates random user data (ideal to test your applications or fake your 10K firsts users) and provides an access to get this data as JSON
If you still haven't installed JSONView, do it now. Here are the links for Chrome / Firefox.
The .getJSON()
method can take the url of a service that sends JSON instead of the path to a .json
file, from this we could access the data of a generated user this way:
$.getJSON( "https://randomuser.me/api/", function( myDataset ) {
var user = myDataset.results[ 0 ];
console.log( user.name.first, user.name.last );
} );
From its documentation, Random User allows us to request multiple users at once, let's try that:
And that's the meaning of adding dynamism to web pages using JS!
I used a few new CSS properties here, you can always look for them on MDN CSS Properties Reference.
All the methods we've seen in the previous examples are just a very small subset of what jQuery can do, so now that you get a bit of understanding on how jQuery works or what it can or might be able to do, I'm going to give you a protip: developers don't need to remember every single methods of each libraries they use, there are hundreds of thousands of CSS Frameworks and JS libraries and they all keep evolving. Instead, and that's the key, we need to learn how to read documentation.
Reading documentation
We're approaching the end of the first part of this course and will soon explore new libraries/methods, there are a few things to consider: whether for manipulating DOM like jQuery, doing animation, creating generative visuals, working with spreadsheets, visualizing data, making interactive music, or anything else, there is a JS library for it. Google something like "JS library 3D modeling", open the result that seems most promising, look at the examples, check the documentation, maybe the number of stars if it's on GitHub to get an idea of the popularity of the lib, and you're ready!
Let's have a look at jQuery's documentation and more particularly to the .css()
method we've already used:
This is an iframe, you can scroll in it instead of going back and forth between tabs.
For a new method, we need to understand how to use it. Try to answer the following questions:
- Read its description (the name of the method should indicate what it does).
-
Look at the arguments the method needs: does it need arguments? How many? For each argument, what should be its type? A string? A number? An object? An array? In some documentations like Console.log() on MDN, you may find something like
console.log( msg [, msg2, …, msgN ] )
, this means that arguments between[ ]
are optional. -
Does the method return something? If yes, what is its type? A string? A number? An object? An array? Sometimes you might encounter
returns void
, this means that the method does not return anything. - Look for examples on how it is used (you can copy the code of the example in a new glitch project and tinker it for a better understanding).
And before using a library in your projects, there are also a few things to consider. Let's have a look at Sweet Alert, a library that makes popup messages easy and pretty.
Have a look at Sweet Alert Documentation in the iframe above, and search answers for the following questions:
- What is the general field of application of the library? Does it solve our problem?
- Are there simple/understandable examples?
- Is the documentation clear? Readable? Does it provide code example for each methods?

The important thing is not to remember every single method, but the ability to find tools for your needs, and to understand how you can work with them.
Sum up
- Libraries are sets of specialized code for a given field
- To use libraries, we need to load them before using them
- Good libraries come with great documentation. If the doc is not clear search for another library
- We can look for and tweak simple examples/snippets to learn from them
- Using the Console and
console.log()
is useful to keep track of what our code is doing
Resources & going further
- Intro to jQuery, Manipulating Websites with Ease
- jQuery Tutorial on w3schools
- jQuery Learning Center
- jQuery by the Examples
- 33 JavaScript libraries and frameworks to check out in 2018
Quiz
Test your knowledge
This quiz is mandatory. You can answer this quiz as many times as you want, only your best score will be taken into account. Simply reload the page to get a new quiz.
The due date has expired. You can no longer answer this quiz.
JS Project
JS Assignment
This assignment is mandatory. If you update your work but the link doesn't change, you don't need to re-submit it.
On Glitch, make sure you're always connected when you make your assignment!
Assignments done without being connected are graded 0!
This project represents your mastering of Part 1: basics, that is to say the end to between 50 and 60 hours of work (1 ECTS = 25/30 hours of work).
Instructions
Remember your manager from the last assignment? They have a new job for you:
Hey 👋
Thank you for the webpage! Looks great. Unfortunately, the data has changed a little bit… and there's a lot more now too.
Here's the new data as a JSON file (will open in a new tab): [Not loading? Please reload the page]
Bummer… did all your work go down the drain? Of course not! Now that we've learnt how to load and display data dynamically using jQuery, we will simply update our previous piece of work. We will keep the styling and simply create all elements using JavaScript!
Here are a few steps to start this assignment:
- To begin with, duplicate (remix) your previous project that you did for assignment 1, in the Design Resources chapter.
(To remix of project of yours, open it in the editor, click on its name in the upper-left corner, and hit "Remix project".) - Then, in your newly created project, create a JavaScript file and name it however you like (for instance,
main.js
). - To have your JS file be loaded in your webpage, don't forget that you have to add a
<script>
tag just before the end of your<body>
tag in your HTML file. - Finally, remember that we're going to use jQuery for this exercise. Therefore, you want to also add a
<script>
tag for jQuery.
Here's what the end of your HTML file should look like:
<body>
<!-- Your content goes here -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<script src="main.js"></script>
</body>
Because our main.js
script is using jQuery, we need to load jQuery first, and our custom script second.
Have a look at the example below to see it all together.
Your project should: To validate, your project must:
- use the dataset that was provided to you
- combine HTML, CSS, and JS
- load data using
$.getJSON()
- create HTML elements using at least 2 pieces of information from the JSON data
Think about Facebook: every time you open facebook in your browser, the page loads data to get your feeds, informations are extracted from this data to create posts with your friends names, their content, number of likes…
The goals of this project are:
- to understand that our pages can load data from other services that serve dynamic/generated/random data.
- to exploit the data-literacy acquired during this part: being able to navigate through complex data structure and extract informations, and that we can think about data in terms of model instead of its values (a name is a name whatever its value, it will be rendered the same).
- to write some JS code using a library, we understand basic promgramming concepts and can use tools and build upon the work of others.
Evaluation criteria
Participation: 4 points
Time spent on course + interaction with resources / examples
Use of JSON: 6 points
Ability to use JSON data and its integration
HTML quality: 5 points
Use of right HTML elements + code clarity
CSS quality: 5 points
Use of Skeleton's grids and classes + custom CSS, use of resources (integration of medias,images/videos/sound, Google Fonts…)
Going further: bonus points
Filter and/or sorting data, provide a way to manipulate the data through UI elements (buttons, inputs, etc.)
Resources
If you need more informations regarding the $.getJSON()
method, you can consult the following links:
Note on $.each( array, function(){ … } )
:
$.each( json.data, function( index, gifObject ){
console.log( gifObject.images.downsized.url );
} );
// is equivalent to the following for loop:
for( var index = 0; index < myDataset.data.length; index = index + 1 ) {
var gifObject = myDataset.data[ index ]; // create a reference Object for the element in myDataset.data at index i
console.log( gifObject.images.downsized.url );
}
Troubleshooting
Oh no, something's not working! Don't worry, here are a few frequent questions and answers, and tips on how to solve your issues. Click on a question that you have to see the answer.
You always want to open your project in another tab (in the editor, in the top-left corner, click on "Show" → "In a New Window"). Here, you want to work with your console open. The console will show errors if something's wrong with your code.
Q: How do I open the console again?
Ctrl
+Shift
+J
for Windows, or Cmd
+Opt
+J
(Mac)
Ctrl
+Shift
+K
for Windows, or Cmd
+Opt
+K
Mac
Q: My website shows the file structure of my project instead of my actual webpage, what's wrong?
Make sure you have a file called index.html
in your project folder.
Q: The console has an error saying
This means that you are trying to access a variable that doesn't exist. See the following example for details.(something) is undefined
, what does that mean?
var person = {
name: {
first: "John",
last: "Doe",
},
age: 42,
}
console.log(person.first); // undefined (that would be person.name.first)
console.log(person.job); // undefined (that key doesn't exist here)
Q: The console has an error saying
This means that you are trying to access a property from a variable that doesn't exist. See the following example for details.cannot read property (something) of undefined
, what does that mean?
var person = {
name: {
first: "John",
last: "Doe",
},
age: 42,
}
console.log(person.nam.first); // error (we made a typo: person.nam is undefined, and we cannot access the property 'first' of undefined)
Q: Okay, so where in my code does the error come from?
In the top-right corner of the error message in your console, you can see the line number where the error is. For instance script.js:13
means that the error is on line 13 of your script.js
file.
Q: Help, nothing shows up in my console! Did I do something wrong?
If you have no error in the console, that's a good sign. However if you have a console.log()
statement somewhere in your JS script and it doesn't show, that could be for a few different reasons.
console.log()
statement is inside of a condition that is never evaluated as true
, or a loop that won't startconsole.log()
statement is inside of a function that is never called.index.html
file.
Q: Things are okay in the console but won't display in the page, what am I missing?
Make sure you're using jQuery's .append()
method to inject content in your page. Mind the selector that you're using, for instance $('.container').append(something)
will only work if you have an element in your page with the class container
.
Q: Oops, the elements that I try to display in the webpage actually show multiple times.
There are a couple reasons why this may happen:
.append()
method on a class selector (for instance $('.container').append(something)
) and there are multiple elements that share that class in your document. jQuery will select all elements with that class. To fix this, you should change the selector and rely on another class, or maybe an ID.
Q: How do I get multiple Skeleton columns side by side with a loop?
This is actually harder to do that it sounds. If you're lost, forget it and display each element below one another (use columns inside each element instead). However, if you're feeling brave, you can use this approach:
i++
in the loop), you could loop on them two by two or three by three for instance, depending on how many columns you want (with i = i + 2
or i = i + 3
in your loop).
.row
class), which will contain your columns.
[i]
for the first column (on the left), then at index [i + 1]
for the column on its right, etc. Make sure to use a condition to check that elements at index [i + n]
actually exist in the array, otherwise at some point you will overshoot the length of your array, and you'll probably end up with an error.
Q: My project froze, I can't seem to open it again?
Two possible reasons:
index.html
file and in your JS script.
If none of these helped you solve your issue, feel free to ask on Slack.
Finally, here are a few things to watch out for:
- It's spelled
length
, notlenght
😉 - Also, it's spelled
.html
, not.htlm
! - Skeleton's grid system relies on a 12-column grid. Make sure that the size of your columns add up to 12, and that they're the only direct children of an element that has the
.row
class.
The Developer Tools panel is your best friend for this assignment. If your layout seems wrong, head over to the "Elements" tab to inspect the DOM (the structure of your HTML code). Open/close elements in that panel to find out the structure that you have, it's often more practical than the actual code in the editor.
You can also inspect the styling rules that are applied to your elements, edit them, or toggle them to see what they do.
Submit your assignment
When sharing your Glitch projects, copy the link for the result (not the editor view). Then, simply paste that link in the submission box down below. 👇
The due date has expired. You can no longer submit your work.
And that's it for the first part! Congratulations, you're doing great!
We've covered the basics of the different languages used for designing the Web.
It is now time to take a step back from these technical skills and to start reading the second part of this course.
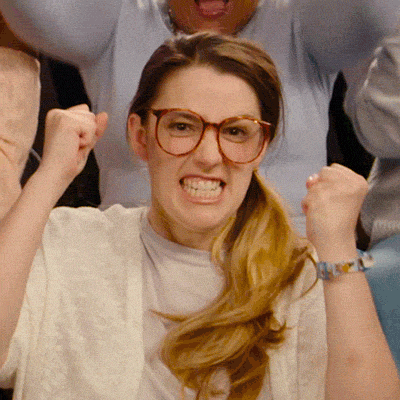