JS - Arrays
Designing with Web
["arrays!"]
What are arrays?
Arrays are a data structure to store a list of values inside a single variable.
To store an array of values inside a variable, we simply use square brackets []
around values separated by commas ,
:
var myArray = [ 5, 1, 12, 13 ];
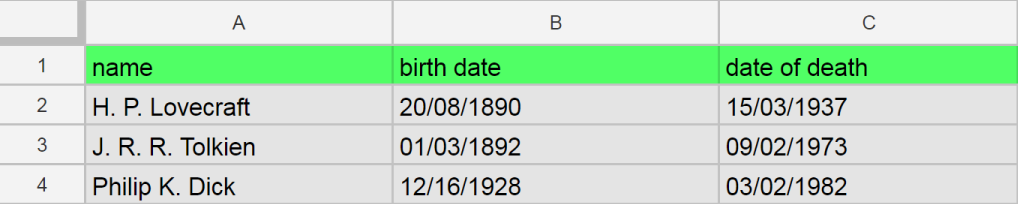
Remember our table of authors from Structuring Data ? We're going to use arrays to store all of it inside a variable:
You can take a look at another way of representing an array. Since an array is simply a list of values in sequence, to access the array's value, you simply have to select it's index. In our case, we use the array's elements to create our circle.
It is common to use repetition loops to walk through all the values in an array:
Multiple Dimensions
We've represented one line of our table as an array, we will now create an array that contains all the data of our table where each line is an array: It is common to have arrays inside an array, this is called a 2D array. Sub-arrays are separated by commas, just like values in an array:
We could as well have more dimensions (more levels of arrays inside arrays): for each authors, add a 4th element which is an array containing book titles, and try to access these values, ie for Lovecraft, add: [ "The Rats in the Walls", "The Call of Cthulhu" ]
Methods
We've seen we can get the number of elements in an array using its length
property: myArray.length
. This syntax is called dot-notation. Using this kind of syntax we can also access methods to dynamically add, remove, search, sort or filter elements.
JS Arrays have a lot of other methods, like filter
that applies a test on each elements and keep in an array only those where the test evaluates to true
; read about these methods on Array on MDN.
Sum up
- Arrays are a way to store multiple values inside a variable.
- Arrays can contain different types.
- We can access values in an array using their index.
- Arrays are zero-indexed.
- We can use loops to loop through arrays.
- Arrays have methods to work with data dynamically.
- We can nest arrays.
Quiz
Test your knowledge
This quiz is mandatory. You can answer this quiz as many times as you want, only your best score will be taken into account. Simply reload the page to get a new quiz.
The due date has expired. You can no longer answer this quiz.
Arrays are an helpful way to store lists of elements, but navigating by index can be difficult if its elements represent different concepts:
-
In an array that represents a list of authors, it makes sense that for
authors[ 0 ]
,authors[ 1 ]
… each represent one author. -
In an array that represents one author,
author[ 0 ]
represents a name,author[ 1 ]
represents a birth date… this can be confusing to associate an index with a concept.
We're now going to look at another way to structure data that will simplify this: Objects.
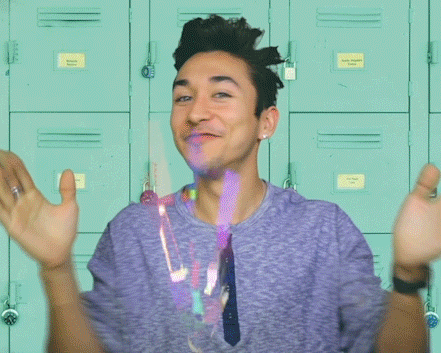