JS - Objects
Designing with Web
{ everythingIs: "an object!" }
What are objects?
Objects can represent real life object in a meaningful way, e.g. an author can have properties like name and birth date, and methods like write, say hello.
In the example below, we have an object called 'circle' which has two properties: size
and position
. You'll notice that position
is an array with two values. As we saw in the previous chapter, those values are accessible by selecting their indexes.
Our author's name is stored in a property name
inside an object that represents our author, which is more meaningful than previously author[ 0 ]
with our Array of authors.
Try to create a new property and access it.
As usual: spaces and line breaks are not important.
Methods
A key that stores a value is called a property, but a key can also reference a function instead: we call it a method, it describes an action that our object can do:
The JS language comes with built-in objects like the Math object that brings constants like Math.PI
or methods like Math.round()
to round a value, or Math.random()
that returns a random value between 0 and 1.
The console.log()
we've been using should be more understandable now: the browser provides us access to a global object console
which has a method log
, to which we pass parameters
to be printed.
Objects and Arrays
Objects properties can of course store arrays:
And we can as well have arrays of objects, for example here our list of authors from Structuring Data:
Finally, here we have an array containing objects containing properties containing objects… Each concept we've seen until now is just a brick than can be reused in other bricks (like conditions inside of loops inside of functions…).
JSON (JavaScript Object Notation) is also widely used to transfer data between web services. Install JSONView (for Chrome / Firefox), then have a look at this JSON data. This is an array of objects representing persons, let's say we have a variable that's equal to this data: var persons = data;
, we can access the name of the first friend of the 2nd person using:
persons[ 1 ].friends[ 0 ].name
(remember zero-indexed arrays).
Navigating through complex data structure and formatting data into models may require a certain mental gymnastics, yet this is a much required skill.
OOP Basics
In our last example, our array of authors, a lot of code was written exactly the same several times. This was only the methods, but it could have been properties like eyes: 2
, legs: 2
, … everything they have in common. We are now going to have a look at a last concept that can seem very abstract but is essential to start projects on your own: Object-oriented programming.
Instead of defining all our objects one by one, we're going to define an Author
constructor that will serve as a model to create our individual authors. This will serve as a base for creating an Author prototype with properties and methods. Finally, we'll create instances, objects modeled on our prototype.
But first we need to look back to a statement that was made in the Variables module: "Everything in JavaScript is an object".
For example, we've seen that arrays have properties (like length
), and methods: Arrays are Objects. But Strings also are objects with properties and methods:
And functions are objects too:
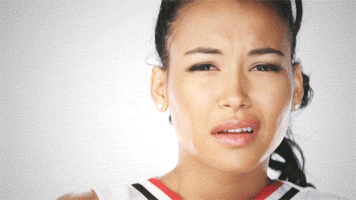
Yes, this is mind bending.
But based on that knowledge, and the this
keyword, we can now create a constructor function, then create as many instances as we need based on that.
We can now quickly create as many authors as we want, based on our constructor, using the new
keyword, with methods and properties having unique values.
Creating objects based on a constructor is only a short introduction to OOP; to get a deeper understanding you should read Object-oriented JavaScript for beginners.
A script inside a web page has access to a built-in instance of the Document class: document
.
document
is a particularly interesting object because it is a JavaScript representation of our HTML document:
-
we can use
document.body
to select the body of our document -
we can search for an element using CSS selectors with the method:
document.querySelector( "#main-title" )
-
we can create elements dynamically with
var myTitle = document.createElement( "h1" );
-
and all elements created or selected are instances of the Node class with methods and properties like:
myTitle.innerText = "my awesome title";
-
and we can add them to other elements:
document.body.appendChild( myTitle );
Sum up
- In JS, everything is an object.
- Objects allow to store complex data structure.
- Objects can have properties (values) and methods (functions).
- We can access properties and methods using dot-notation.
- Inside an object, we can use the
this
keyword to refer to itself. - Using a constructor as a model, we can create uniques instances.
Resources & going further
- JavaScript for Cats
- JavaScript by the Examples
- Eloquent JavaScript
- You don't know JS
- Intro to JavaScript, Fundamentals of the JavaScript Syntax on Udacity
Quiz
Test your knowledge
This quiz is mandatory. You can answer this quiz as many times as you want, only your best score will be taken into account. Simply reload the page to get a new quiz.
The due date has expired. You can no longer answer this quiz.
Congratulations!
You've gone through all the basic concepts and are now ready to use all this JS knowledge with our websites! In the next section, we're going to explore an awesome aspect of JS: Libraries.
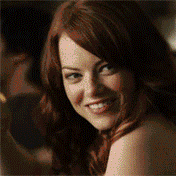