JS - Conditions
Designing with Web
Yes no, maybe, I don't know…
What's a condition?
In any programming language, code needs to make decisions and carry out actions accordingly depending on different inputs. For example:
- in a game, if the player's number of lives is 0, then it's game over.
- in a weather app, if it is being looked at in the morning, show a sunrise graphic; if it is night-time, show stars and a moon.
In this chapter, we'll explore how conditional structures work in JavaScript.

Conditions are code structures which allow you to test if an expression returns true or false, running alternative code revealed by its result. We can compare values using comparison operators.
Comparison operators
Equality ===
Tests if two values are equal.
console.log( 10 === 10 ); // true
console.log( 10 === 20 ); // false
Inequality !==
Tests whether two values are not equal.
console.log( 10 !== 10 ); // false
console.log( 10 !== 20 ); // true
Greater than >
Tests whether the left operand is strictly superior to the right operand.
console.log( 10 > 5 ); // true
console.log( 10 > 10 ); // false
console.log( 10 > 20 ); // false
Greater than or equal >=
Tests whether the left operand is greater than or equal to the right operand.
console.log( 10 >= 5 ); // true
console.log( 10 >= 10 ); // true
console.log( 10 >= 20 ); // false
Less than <
Tests whether the left operand is strictly inferior to the right operand.
console.log( 10 < 5 ); // false
console.log( 10 < 10 ); // false
console.log( 10 < 20 ); // true
Less than or equal <=
Tests whether the left operand is less than or equal to the right operand.
console.log( 10 <= 5 ); // false
console.log( 10 <= 10 ); // true
console.log( 10 <= 20 ); // true
There also exist ==
and !=
operators, but they are not recommended, see Equality comparisons and sameness.
We can also compare strings using these operators: console.log( "Bob" !== "Steve" ); // true
Strings will be compared on their alphabetical order for relational comparisons (>
, >=
, <
, <=
)
if statement
Now that we know how to compare 2 values, let's get back to conditions. We're going to use the if
keyword to check the result of a comparison and choose what instructions to execute:
We can also use an else
statement to add instructions in case our condition is evaluated to false
:
You will find below an interactive illustration of a simple if… else
conditional. If the mouse position is on the left part of the screen, we return true
, otherwise it's false
.
The expression inside the if ( … )
is the test: this uses the identity operator (as described above) to compare the variable iceCream
with the string chocolate
to check if the two are equal. If this comparison returns true
, the first block of code is run. If the comparison is not true
, the first block is skipped and the second code block, after the else
statement, is run instead.
if… else if statement
The if… else if
statement is an advanced form of if… else
that allows JavaScript to make a correct decision out of several conditions.
We can also have nested conditions, actually the previous example could have been written like this:
switch statement
We can use multiple if… else if
statements to perform a multiway branch. However, this is not always the best solution, especially when all of the branches depend on unique values of a single variable. We can use a switch
statement which handles exactly this situation, and it does so more efficiently than repeated if… else if
statements.

Like else
, default
case is optional, we can choose to treat only some case and do nothing if conditions are not met, i.e. false.
Sum up
- We can use conditions to take decisions with code.
- Conditions are based on comparisons that evaluate either to
true
orfalse
. - Comparisons are made using comparison operators.
- We can use single case/multiple cases conditions, using
if
,else
,else if
orswitch
- We can set default instructions in case all our tests are
false
usingelse
/default
Logical operators can also be used to test combinations of conditions:
-
AND:
condition1 && condition2
, both conditions need to betrue
-
OR:
condition1 || condition2
, at least one of the conditions needs to be true
Quiz
Test your knowledge
This quiz is mandatory. You can answer this quiz as many times as you want, only your best score will be taken into account. Simply reload the page to get a new quiz.
The due date has expired. You can no longer answer this quiz.
Now that we've seen variables and conditions, every other programming concepts will allow us to write less code, being more concise. Let's move to Repetition Loops.
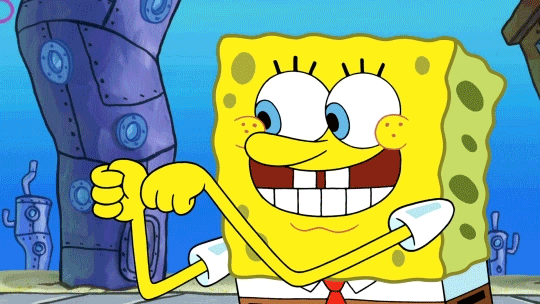