JS - Loops
Designing with Web
Loops! Loops! Loops!
What are loops?
Sometimes you need a task done more than once in a row. Loops are all to do with doing the same thing over and over again, which is termed iteration in programming speak. After maths, this is another thing computers and programming are great at, repeating again and again.
In programming, we should always try to follow the DRY principle: Don't Repeat Yourself. So, instead of repeating our code over and over, we'll see how to use loops.
Let's say we have 3 tasks we want to accomplish today before doing anything else, we could write the following program:
This seems ok, but what if i ask you to do 100? Maybe using a variable and copy paste:
Well thought, but still painful to write, to read and to maintain. That's where loops come in handy, they avoid us to repeat code. Let's say we've already done 3 tasks done out of 100. We're going to describe what our loop needs to do for us:
- Right now, we've done 3 tasks; this is our starting point (called initializer):
var tasksDone = 3;
-
Until we have our 100 tasks done, we're going to repeatedly (this is our condition):
while( tasksDone < 100 )
- do what we need to accomplish one task (this is our list of instructions)
- add 1 task to our list of tasks done (this is our final-expression):
tasksDone = tasksDone + 1;
- go back to our condition
- If we have 100 tasks done, we get out of the loop, and continue our way.
All loops have these 4 parts: initializer, condition, list of instructions and final-expression. We're now going to write this with code using 2 different loops, while
loops and for
loops. Loops are based on variables and conditions in addition to these new keywords.

While loops
while
loop syntax is very close to a single if
condition, with the 4 elements we discussed above:
initializer
while ( condition ) {
list of instructions
final-expression
}
Applied to our tasks, we can write our while
loop this way:
We've reduced 400 lines to 10, quite efficient. And the syntax of while
loops is understandable.
Try to modify the different parts of the loop, like modifying the initializer (i.e. var tasksDone = 67;
), modifying the condition (like tasksDone < 124
) or increasing the incrementation in the final-expression (tasksDone = tasksDone + 5;
).
While this is a simple program with a few instructions, the problem with while
loops is that the elements that control our loops (initializer, condition, and final-expression) are scattered across the program, that's why developers tend to use for
loops instead.
For loops
for
loops syntax is more concise:
for ( initializer; condition; final-expression ) {
list of instructions
}
And applied to our tasks, we can write our for
loop this way:
While it may seem more obscure, once used to it, its concision makes it easier to read and less prone to error since the elements that control our loop (initializer, condition, and final-expression) are in the same place.
Play with the example down below to observe how changing the for-loop's elements can change the results.
Sum up
- We can repeat instructions using loops.
-
Loops need 4 elements:
- an initializer: when the loop should start
- a condition: when the loop should stop
- a list of instructions: what instructions should be repeated
- a final-expression: how do we get to the next repetition
- We can use the variable inside the loop to affect instructions.
There are several other loops in JS, but they are hardly used, you can find them all here on Looping Code.
Quiz
Test your knowledge
This quiz is mandatory. You can answer this quiz as many times as you want, only your best score will be taken into account. Simply reload the page to get a new quiz.
The due date has expired. You can no longer answer this quiz.
That's it for loops. We can now move on to Functions.
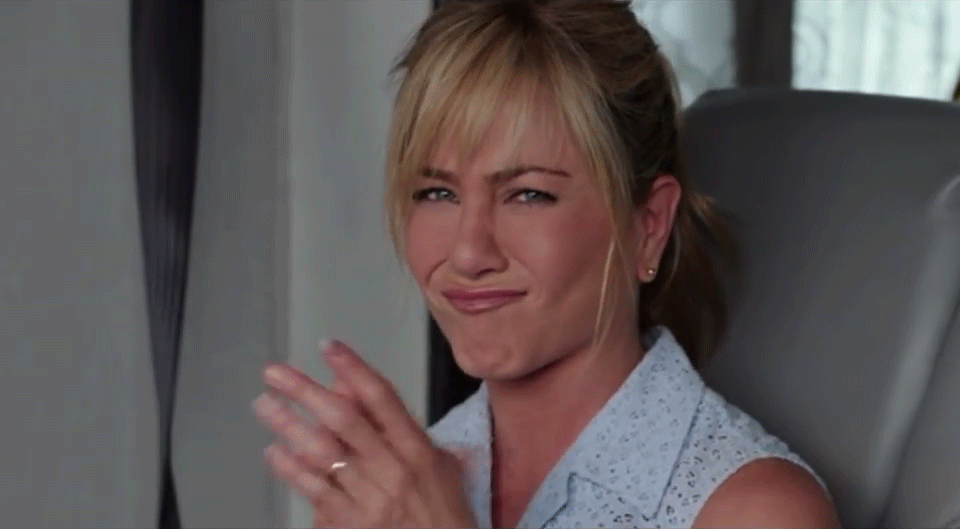