JS - Functions
Designing with Web
sayHi("to functions!");
What are functions?
Functions are a way of packaging a list of instructions that you wish to reuse, you can think of it like a recipes: a list of instructions to follow in a certain order, that you can execute whenever you need.
We've already used a few functions like:
alert( "hi" );
console.log( "hello" );
These functions, alert
and console.log
, are built into the browser for us to use whenever we desire, and execute a list of instructions to create a popup or print a message in the console. We can create our own functions using the function
keyword, followed by a name we choose to describe what our function will be doing, plus parentheses ()
, and finally brackets { … }
that will contain the instructions we want to package:
To execute our function, we need to call its name (like variables) plus parentheses ()
.
Uncomment line 8 (greetBob();
) to invoque its execution.
The names of our functions follow the same naming rules as variables, for a simple reason:
function myFunction() { … }
is an abbreviation for var myFunction = function() { … }
Until now, the lecture of our scripts was linear, executing instructions line by line. By using functions, we can organize our code in blocks of instructions and reference them when we need to. For example:
function firstFunction() {
console.log( "first function" );
}
function secondFunction() {
console.log( "second function" );
}
firstFunction(); // execute all the instructions in the first function
secondFunction(); // then execute the second
function firstFunction() {
console.log( "first function" );
secondFunction(); // execute the second one, from the first one
}
function secondFunction() {
console.log( "second function" );
}
firstFunction(); // call the first function
In the first example, we call 2 functions one after the other. In the second example, the call to secondFunction
is made inside firstFunction
. Both examples produce the same result, but in the first one we can choose to execute our functions separately, while in the second, secondFunction
will always be called when we execute firstFunction
(which is ok if this is what you need). This is up to you to organize your code according to your needs.
Arguments
Functions often take arguments, these go inside the parentheses. For example, the alert()
function makes a pop-up box appear inside the browser window, but we need to give it a string as an argument to tell the function what to write in the pop-up box. Using arguments is another way to re-use parts of our code. It's like having parameters that would slightly affect the result, e.g. an omelette recipes where you put 5 eggs instead of 3. Let's review our previous greeting function and add an argument to it:
The argument name
is the same as a variable that takes the value used when our greet
function is called: "Emma"
for the first call, "Harry"
for the second, …
The arguments of a function can only be accessed inside this function, try to print name
after the function: console.log( name );
Below you'll find a visual example of a function's behavior. Two arguments called "x" and "y" are passed into drawWhiteCircle which will use them to create a circle at mouse position.
We can also use conditions and loops inside functions:
Functions can take more than one argument, arguments have to be separated by commas ,
:
As an example, we're going to write a simple function which takes two numbers as arguments and multiplies them:
Arguments take their values in the order they are specified: firstNumber
always takes the first value, secondNumber
always take the second value.
We can now use arguments to parameterize our functions and print the result of our functions but what if we want to use the result of our functions, we need one more statement to actually use the result outside of the function: this is the return
statement.
Return
The return
statement tells the browser to return the value of the variable result
so this value can be assigned to a variable outside of the function. This is necessary because variables defined inside functions are only available inside those functions. This is called variable scoping. (Read more on variable scoping).
The return
statement must be the last instruction of your function, since it makes the code flow escape of the function.
Add console.log( "test" );
on line 4 and observe if it is executed.
Callback functions
We've seen we can pass whatever we want to a function as an argument, we then need to treat our argument according to what it represents (i.e. doing multiplication if our argument is a number, doing string manipulation if it contains a string…).
We can even pass a function as argument:
Using functions as arguments is particularly useful when we want to execute a function at a given time, or once a function is completed, or at a particular interaction:
Click on the View App
button and test the program.
Sum up
- Functions are a way to package instructions.
- Functions make code more re-usable.
- Functions can take arguments.
- Arguments of a function can be used only inside this function due to scope.
- Variables created inside a function can be used only inside this function due to scope.
- Functions can return a value.
- Functions can be passed as arguments and are called callbacks.
Quiz
Test your knowledge
This quiz is mandatory. You can answer this quiz as many times as you want, only your best score will be taken into account. Simply reload the page to get a new quiz.
The due date has expired. You can no longer answer this quiz.
And that's it for functions! The next and last 2 elements we're going to see now will bring us back to the beginning of this course and structuring data, let's start with Arrays.
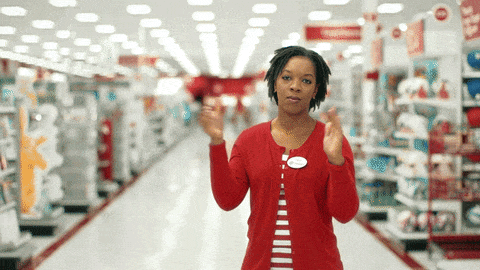