Styling the Web
Designing with Web
Different language, different purpose, different syntax
What is CSS?
Cascading Style Sheets (CSS) is the language we need to style your webpage. Like HTML, CSS is not a programming language, it is not a markup language either, it is a style sheet language. This means that it lets you apply styles selectively to elements in HTML documents using rules.
Browsers have default styles (which can be different depending on the browser) for rendering each HTML element. We can overwrite these styles by adding our own CSS rules.
For example, to set the text color of paragraphs to red, we can write the following rule:
CSS uses selectors to define which elements are going to be applied a set of rules delimited by brackets { … }
: here p
selects all the paragraph elements of our HTML document. A rule is composed of a property (here color
) followed by a :
(colon), then the value to be applied (here red
which is a recognized as a valid color value) and finally a ;
(semi-colon).
Try to write a new rule to set the text color of the h1
element to green
.
It is common to forget a ;
or an }
, Glitch signals errors with red circles on the left of your code, that you can hover to get informations. You can also use W3C's CSS Validation Service to get more informations on how to fix your CSS.
Before writing styles to our HTML elements, let's have a quick look at how we can target specific elements.
Selectors
We've seen in the previous examples that we can use the tag name of elements (like p
or h1
) to apply CSS rules to all the elements with the same tag name in our HTML doc, this is the type selector:
Type selector
Selects all elements that match the given tag name.
- Syntax:
tagname
- Example:
img
will match all the<img>
elements.
But what if we want to select only some of them? Or just one? There are different ways to select HTML elements using their attributes. For example, we can use the ID selector to target only the element with a specific id
attribute, or the class selector to select all the elements with a specific class
attribute.
Here are some of the basic selectors:
ID selector
Selects an element based on the value of its id
attribute.
Syntax: #idname
Example: #solution
will match the element with id="solution"
.
Class selector
Selects all elements that have the given class
attribute.
Syntax: .classname
Example: .important
will match all the elements with class="important"
.
Attribute selector
Selects elements based on their attributes.
Syntax: [attr]
or [attr=value]
Example: [href]
will match all elements with a href
attribute (set to any value).
Universal selector
Selects all elements.
Syntax: *
Example: *
will match all the elements of the document.
ID's are unique:
- Each element can have only one ID
- Each page can have only one element with that ID
Classes are NOT unique:
- You can use the same class on multiple elements
- You can use multiple classes on the same element
Check The Difference Between ID and Class for more background.
We can also combine multiple selectors
by separating them with a coma ,
:
h3, #header, .red-text {
color: red;
}
Our rules will be applied to all the h3
elements, as well as the element with the id header
, and all the elements the red-text
class. You can check the complete list of selectors on MDN if you need to target elements with more complex rules, like direct children of an element, even elements in a list, or to change the style of an element when your mouse is over it.
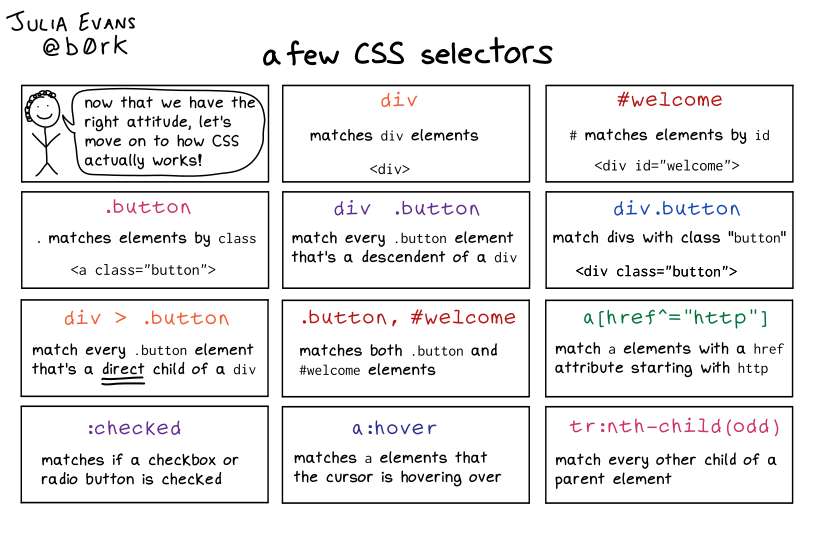
Now that we've looked at how to target elements using selectors, let's style them!
Rules
We can do a lot of things using CSS from styling colors, backgrounds, typographies, spacings, sizes, margins, positions, shadows, rounded corners, layouts… of our elements. Even animate them:
Click on the HTML
and CSS
buttons to take a look at the source.👆👆👆
CSS rules are the key point to go from the elements we wrote in the HTML section with their default styles, to how they look in the different websites we use everyday. Have a look at google.com with or without CSS:

Actually, there are so many CSS properties we can modify on elements (have a look at the CSS Properties Almanac), that instead of flooding you with tons of rules, it will be easier to learn from examples as we continue this course.
So, how to use CSS?
To use CSS, we're going to use external stylesheets: we need to create a .css
file (like we did to create an index.html
), this new file will store our CSS rules. Here, I created a style.css
file:
This CSS file contains the rules for styling the elements in our index.html
(and a lot of comments for you to understand what these rules do). We now need to link this file to our HTML document using a link
element.
Include the following code inside the head
of index.html
:
<link rel="stylesheet" href="style.css">
Once you've linked style.css
to index.html
, click on the View App
button. Then get back to the source and observe the structure of index.html
(like the usage of a div
to center everything, or span
elements, class
and id
attributes) and change the values in style.css
or comment lines to see how it affects our page.
The link
element is an empty element (it does not have a closing tag). The rel
attribute specifies the relationship between the current document and the linked document, and the href
attribute specifies the location (URL) of the external resource. It is common to put link
elements in the head
of HTML documents in order to load the stylesheet before displaying the content of pages, instead of displaying them with default styles and then charging styles.
Inline CSS and Internal Stylesheets
There are other ways to use CSS, but using external stylesheets is considered best practice because we won't have CSS rules all over our HTML document, unlike inline CSS where we use a style
attribute on HTML elements:
With inline CSS we set style for each element individually, thus if we want to change a property, we need to update it everywhere to keep consistency.
Here I am using Lorem Ipsum, a dummy text used for testing layouts. You can learn more about it on loremipsum.io.
Internal stylesheets are another way to use CSS, for this we use a style
element in the head of our HTML document:
It's more convenient than inline CSS since you can set properties to a while seet of elements using selectors, but separating structure (HTML) and styles (CSS) with external stylesheets (.css
files) makes it easier to work with.
Boxes, boxes, it's all about boxes
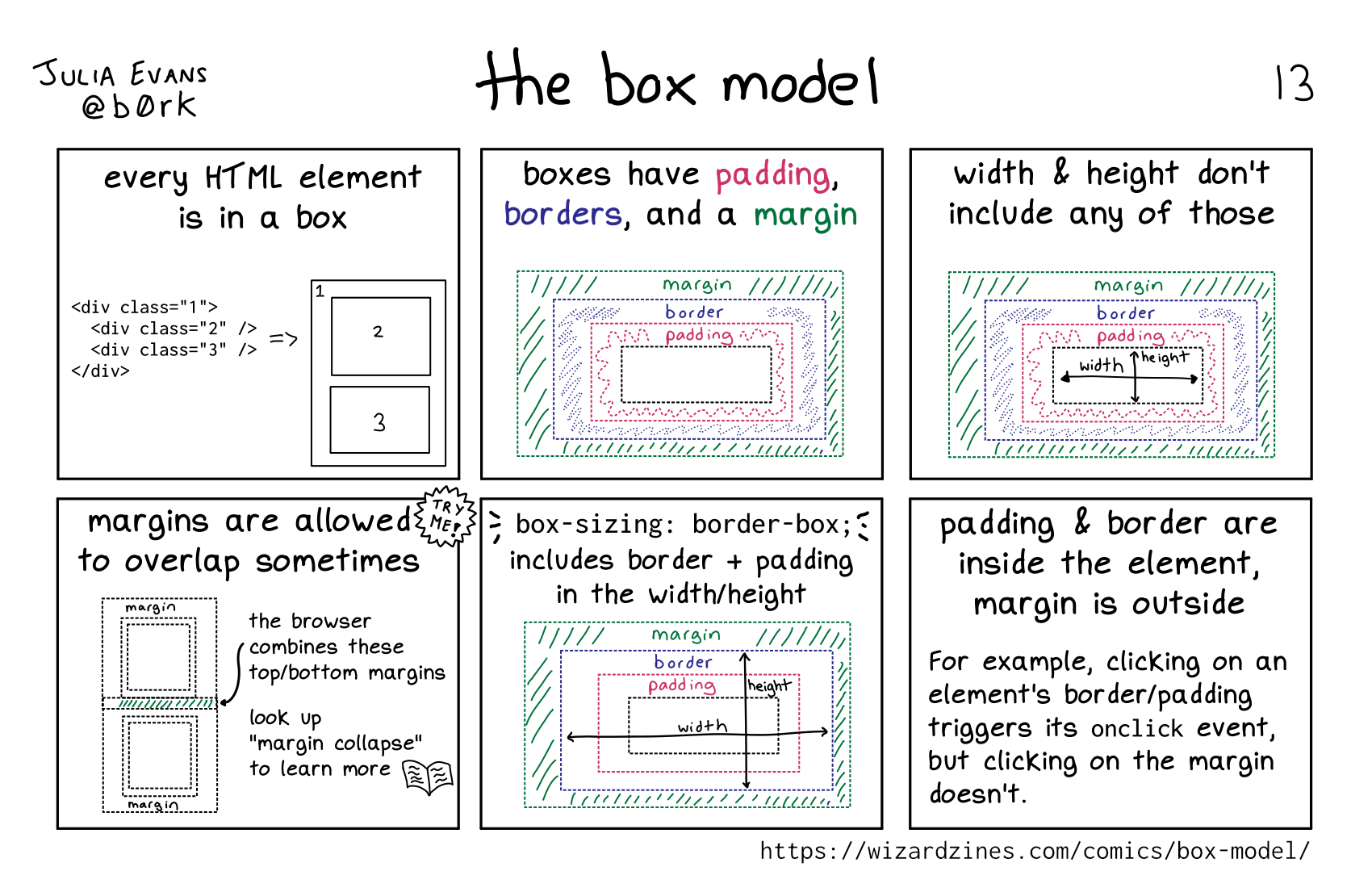
As you may have already noticed from the previous examples, most of the HTML elements on your page can be thought of as rectangular boxes sitting on top of each other. These boxes adapt their height to their content, but behave in 2 ways depending on the type of element:
-
block elements, like headings,
p
,section
,div
, layout elements… always start on a new line and take up the full width of the page: an element following a block element also always start on a new line. -
inline elements, like
strong
,span
,code
,img
… do not start on a new line and only takes up as much width as necessary. They can be followed on the same line by content / other inline elements.
h1
and p
elements have their display
property set to block
by default, while span
elements are inline
by default.
Uncomment line 2, then set display
property to inline
and see how boxes are affected.
There are also other properties to consider with the size of our boxes: margins, borders and paddings.
margin
defines the empty space outside of the element while padding
defines the empty space inside of the element.
We can also define margins separately using margin-top
, margin-right
, margin-bottom
and margin-left
. And it's the same with paddings.
You should read more about the CSS Box Model to better understand how it works.
Some notes on rules
- CSS rules apply to the elements selected and affect their children.
-
Numeric values are (nearly) always followed by a unit (
px
,%
,vh
… ): read CSS values and units and CSS - Measurement Units for more information - If several rules are conflicting (same property with different values), there are some basic rules that a browser follows to determine which one is most specific and therefore wins out. Read more about those rules.
Change the width
of the div
and observe how it affects children p
elements, what color is used for background
on p
elements, the different units used.
Sum Up
- We can use Cascading StyleSheet language to style HTML elements.
- CSS uses selectors to target a specific element or several elements.
- CSS uses
property: value;
rules. - We can combine selectors to apply the same rules to different elements.
- We can write CSS rule for each styling property: fonts, spacings, colors, positions, shadows, borders…
Resources & going further
- For a complete reference for selectors, rule properties and their possible values, check CSS Almanac
- Learning CSS by the examples on w3schools
- Learn CSS on tutorialspoint
- Learn to style HTML using CSS on MDN
- CSS-Tricks: Tips, Tricks, and Techniques on using Cascading Style Sheets
- HTML ans CSS Syntax on Udacity
Quiz
Test your knowledge
This quiz is mandatory. You can answer this quiz as many times as you want, only your best score will be taken into account. Simply reload the page to get a new quiz.
The due date has expired. You can no longer answer this quiz.
Good job, you now have the basics for styling webpages! Yet CSS can be really tedious to keep homogeneity across all our elements, that's why we're now going to have a look at Design Resources.
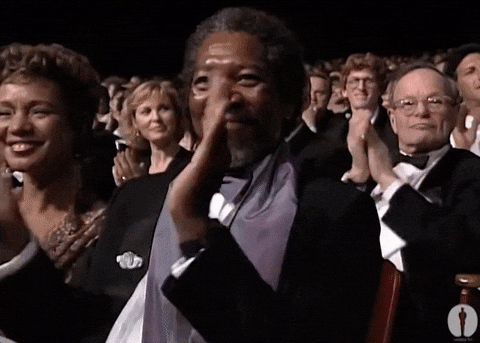